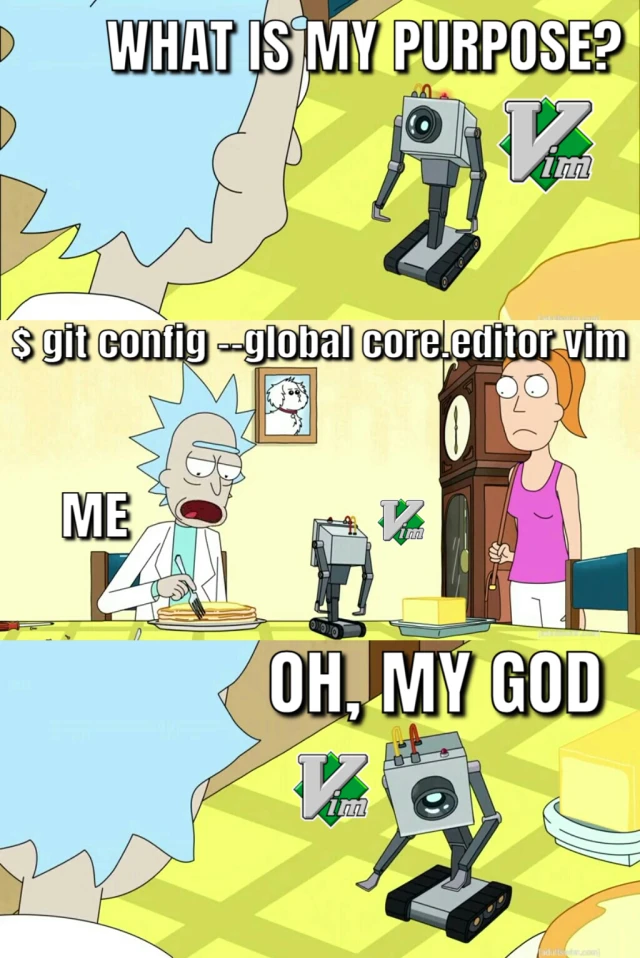
# My Minimal yet Practical vimrc
I used to configuring my vim to make it looks like and also works like an IDE. But after tons of time spent on configuring, investigating those weird nasty problems, finding solutions and workarounds, I realized that a pistol can never be a AK-47 no matter how hard you try to modify and tune it.
IDEs and other proper GUI text editors have all the good builtin features coming out of box, like minimap, file searching, git integration, remote development and language server support.
I use Kate for all my personal side projects, it’s lightweight and fast, it can be used without any freaking configurations. And I don’t use LSP feature of Kate, because I clearly remember what I wrote in my personal not-so-big codebase.
For other things/projects that are I am not familiar with, often in C/C++/Python, I usually use VSCode with LSP, if it’s properly documented or can be configured easily.
However, vim does shine in other ways, for example, based on my experience:
-
Quick editing: The modification is small that it’s annoying to switch to another window or open an editor to do this, like fixing compiler complaints.
-
As the git editor: Writing commit messages, rebasing and squashing commits, editing patches… ── Might be the most useful scene.
-
On some remote server ── Sometimes.
My .vimrc
is aimed at building a minimal, basic and pratical vim, it works for my everyday needs.
syntax on
filetype plugin indent on
" set termguicolors
set encoding=utf-8 " encoding
set background=dark
set nocompatible " no vi compatible
set mouse=a " enable mouse support
set clipboard=unamedplus " X11 system clipboard, but not work for me
set confirm " prompt when exiting from an unsaved file
set noerrorbells " no fucking error bells!
set showcmd
set wildmenu " provide a graphical autocomplete menu for command mode
set lazyredraw " redraw the screen when we need to
" tabs and indent
set tabstop=4 " visual <Tab> width
set softtabstop=4 " editing <Tab> width
set shiftwidth=4 " indent width, used for autoindent, << and >> operators in normal mode
set expandtab " convert <Tab> to spaces
set smarttab
set autoindent " copy indent from current line when starting a new line
set smartindent " better autoindent, add indent after '{' for instance
autocmd FileType * setlocal formatoptions-=c formatoptions-=r formatoptions-=o " disable automatic comment insertion at new line
set number " show line numbers
set relativenumber " show relative line numbers of the cursor
set ruler " show line and column number of the cursor on right side of statusline
" set nowrap " uncomment this if want wrapped lines
" swap and backup
set nohidden
set noswapfile
set nobackup
set nowritebackup
set undofile
" searching
set hlsearch " highlight matches when searching
set incsearch " increment search as characters are entered
set ignorecase
set smartcase
set showmatch " highlighting matching parentheses or brackets
set scrolloff=8 " scroll the screen when 8 lines left
set shortmess+=c
set list listchars=tab:»·,trail:·
" always display status line
set laststatus=2
set noshowmode
" current vim mode
let g:curr_mode_text_map = {
\ 'n' : 'Normal',
\ 'no' : 'Normal·Operator Pending',
\ 'v' : 'Visual',
\ 'V' : 'V-Line',
\ "\<c-V>" : 'V-Block',
\ 's' : 'Select',
\ 'S' : 'S-Line',
\ "<c-S>" : 'S-Block',
\ 'i' : 'Insert',
\ 'R' : 'Replace',
\ 'Rv' : 'V-Replace',
\ 'c' : 'Command',
\ 'cv' : 'Vim Ex',
\ 'ce' : 'Ex',
\ 'r' : 'Prompt',
\ 'rm' : 'More',
\ 'r?' : 'Confirm',
\ '!' : 'Shell',
\ 't' : 'Terminal'
\ }
hi User0 cterm=bold ctermfg=black ctermbg=white
hi User1 cterm=none ctermfg=black ctermbg=gray
function GetModeText ()
let md = mode()
if (has_key (g:curr_mode_text_map, md))
return toupper(g:curr_mode_text_map[md])
endif
return toupper(md)
endfunction
set statusline=
set statusline+=%0*
set statusline+=\ %{GetModeText()}\
set statusline+=%1*
set statusline+=\
set statusline+=%F " full file path
set statusline+=%m " modified flag
set statusline+=%r " read-only flag
set statusline+=%h " help file
set statusline+=%w " preview
set statusline+=\
set statusline+=%2*
set statusline+=%= " switch to right side
" file type, file encoding, line number, total line count, column number, percentage
set statusline+=\ %y\ \ %{&ff}\ \ %{&fileencoding}\ \ %l/%L:%c\ \ %p%%
" don't jump over wrapped lines
nnoremap j gj
nnoremap k gk
nnoremap gj j
nnoremap gk k
" some emacs key combos
" move one character forward
inoremap <C-f> <Right>
" move one character back
inoremap <C-b> <Left>
" important, in order to get the fucking alt key work in konsole
execute "set <A-f>=\ef"
execute "set <A-b>=\eb"
" move one word forward
inoremap <A-f> <C-Right>
" move one word back
inoremap <A-b> <C-Left>
" move to the beginning of line
inoremap <C-a> <C-o>I
" move to the end of line
inoremap <C-e> <End>
" move to the previous line
inoremap <C-p> <Up>
" move to the next line
inoremap <C-n> <Down>
" delete one character forward
inoremap <C-d> <Del>
" delete one character back
inoremap <C-h> <Backspace>
" delete to the end of line
inoremap <C-k> <C-o>D
" delete to the beginning of line (this one it's actually bash)
inoremap <C-u> <C-o>d0
" some bash-like key bindings for command mode
cnoremap <C-a> <Home>
cnoremap <C-e> <End>
cnoremap <C-p> <Up>
cnoremap <C-n> <Down>
" move the current line or a selected block of lines up or down
" the keycodes when pressing <Alt-x> is the same as <Esc-x> in my terminal (konsole)
" so we must first set these two mappings, or <Alt-x> will not work
" actually you should avoid mapping the <Alt-x> key, where x is a letter
" you can use `sed -n l` two check the keycode your terminal is sending to vim
" see :help :map-alt-keys for more information
map <Esc>j <A-j>
map <Esc>k <A-k>
nnoremap <silent> <A-j> :m .+1<CR>==
nnoremap <silent> <A-k> :m .-2<CR>==
" inoremap <silent> <A-j> <Esc>:m .+1<CR>==gi
" inoremap <silent> <A-k> <Esc>:m .-2<CR>==gi
vnoremap <silent> <A-k> :m '<-2<CR>gv=gv
vnoremap <silent> <A-j> :m '>+1<CR>gv=gv
xnoremap <silent> <A-j> :m '>+1<CR>gv=gv
xnoremap <silent> <A-k> :m '<-2<CR>gv=gv
" reselect visual block after indent
vnoremap < <gv
vnoremap > >gv
xnoremap < <gv
xnoremap > >gv
It’s well-documented, almost every line has a comment, and I have some emacs-like keyboard combinations/shortcuts based my own preferences.
Vim mode | Shortcut | Function |
---|---|---|
Insert/Command | Ctrl-A | Move to the beginning of line |
Insert/Command | Ctrl-E | Move to the end of line |
Insert | Ctrl-B | Move one character back |
Insert | Ctrl-F | Move one character forward |
Insert | Ctrl-P | Move to the previous line |
Insert | Ctrl-N | Move to the next line |
Insert | Alt-B | Move one word back |
Insert | Alt-F | Move one word forward |
Insert | Ctrl-D | Delete the next character |
Insert | Ctrl-H | Delete the previous character |
Insert | Ctrl-K | Delete to the end of line |
Insert | Ctrl-U | Delete to the beginning of line |
Normal/Visual | Alt-K | Move current line / visual line / visual block up |
Normal/Visual | Alt-J | Move current line / visual line / visual block down |
Visual | < | Unindent visual line / visual block |
Visual | > | Indent visual line / visual block |

You can try it use the commands below:
mv ~/.vimrc ~/.vimrc_backup
wget https://blog.xack.me/posts/2021/my-minimal-yet-pratical-vimrc/vimrc -O ~/.vimrc
And restore your original setup by:
rm ~/.vimrc && mv ~/.vimrc_backup ~/.vimrc
Until next time!